Razor API
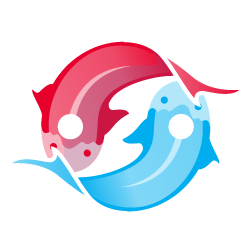
Basic API
Koi.Css : string
- current CSS framework
In most situations this isn't even necessary, but if you want to know what framework is currently used, just ask for Koi.Css
. For example to debug:
@using Connect.Koi;
<span>Currently using the @Koi.Css framework</span>
<span>Currently using the bs3 framework</span>
Koi.PickCss(string list[, string fallback]) : string
- CSS framework if in list
Sometimes you need the CSS framework code - if it's one of the frameworks you support. Here's an example: Your component has css-files for bootstrap3 and 4, and foundation 6. And your strategy is to include the Bootstrap3 version if neither of these matches. The easiest way to do this is using @Koi.PickCss("bs3,bs4,fnd6", "bs3")
which will return either the code "bs3", "bs4", "fnd6" if it's one of those, or "bs3" if it's another or an unknown framework.
For example:
@using Connect.Koi;
@* include bs3 styles if unknown *@
@Koi.IfUnknown("<link rel='stylesheet' href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css'>")
@* include our optimized css file for each scenario *@
<link rel='stylesheet' href='@(Koi.PickCss("bs3,bs4,fnd6", "bs3")).css'>
Koi.IsUnknown : bool
- detect an unknown / undefined framework
If the skin doesn't publish what it has, then Koi.Css
will return unk
for unknown. But in most cases, you'll actually have something in mind - so the most common commands will be:
@using Connect.Koi;
<span>@(Koi.IsUnknown ? "unknown" : "it's known!")</span>
General Html API
@Koi.If(key, trueString [, falseString]) : HtmlString
- choose a value
Use @Koi.If
to return a value (or an alternative):
@using Connect.Koi;
<span>Bootstrap3 status is @Koi.If("bs3", "true", "false")</span>
@Koi.IfUnknown(trueString [, falseString]) : HtmlString
- output if not known
This syntax is used to explicitly insert something if we have an unknown framework - for example, to include Bootstrap3 if we just don't know what's included:
@using Connect.Koi;
@Koi.IfUnknown("<link rel='stylesheet' href='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css'>")
API to create class
attributes
Manually Coding the a Class Attribute (not recommended)
This manual way is included for those wanting more control - later on I'll show the most efficient way to do the same.@using Connect.Koi;
@{
var mainDivClass = "ga-albums";
var innerDivClass = "ga-album sc-element";
switch(Koi.Css) {
case "bs3":
mainDivClass += "row";
innerDivClass += "col-xs-6 col-sm-4 col-md-4 col-lg-4";
break;
case "bs4":
mainDivClass += "row4";
innerDivClass += "col-xs-6 col-sm-4 col-md-4 col-lg-4";
break;
default: // unknown or any other one
mainDivClass = "row";
innerDivClass += "col-xs-6 col-sm-4 col-md-4 col-lg-4";
}
}
<div class="@mainDivClass")>
@foreach (var album in AsDynamic(Data["Default"]))
{
<div class="@innerDivClass">
...
</div>
}
</div>
@Koi.Class(stringFormula) : HtmlString
(recommended)
Now here's the probably easiest way to build such a class attribute:
<div @Koi.Class("all='ga-albums' bs3,unk='row' bs4='row4'")>
</div>
all
frameworks get the class ga-albums- bootstrap 3
bs3
will also get row added - bootstrap 4
bs4
will get row4 added - in case the framework is unknown
unk
, it'll do the same thing as bootstrap 3
Further down you'll see exactly what the syntax looks like.
The keys in @Koi.If(...)
, @Koi.Is(...)
Whenever you check for keys - like @Koi.If("bs3", "it's bs3", "it's not bs3")
the command supports multiple keys. So you can write the following:
@Koi.Is("bs3")
@Koi.Is("bs3,bs4")
@Koi.Is("bs3,bs4,fnd6")
The formula used in @Koi.Class
The QuickFormula is what you'll use most, whenever you use @Koi.Class(...)
. Here some basic examples:
bs3='row-m'
bs3='row-m color5' bs4='row-xs color5'
all='color5' bs3='row' bs4='row-xs'
all='color5' bs3,bs4,unk='row' fnd6='fd-xyz'
all='color5' bs3,bs4,unk='row' bs3='xyz' bs4='abc' fnd6='fd-xyz'
new multi-key support in v1.2
- the formula can contain many parts
- each part has the pattern keys='values'
- keys can be one or more css-framework names, so
bs3
is valid, as well asbs3,bs4,fnd6
- you can also use the keys
all
for parts which should always be included - or
unk
in case the framework is unknown, because the theme doesn't publish the css-framework it uses